Solving the Monty Hall Problem with JavaScript: A Winning Strategy
-
Eric Stanley
- December 22, 2022
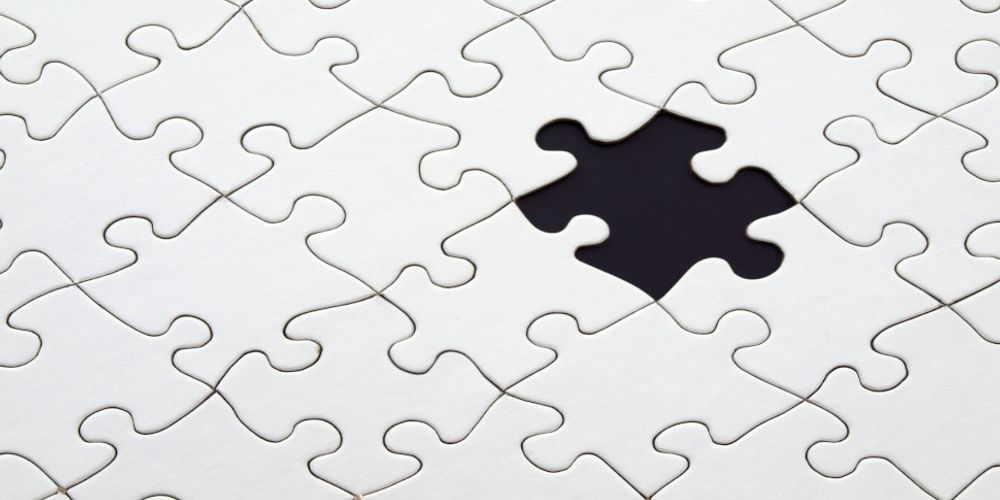
Introduction
The Monty Hall Problem is a famous probability puzzle that originated from a television game show called Let’s Make a Deal hosted by Monty Hall. The problem presents a scenario where a contestant is given the opportunity to choose one of three doors, behind one of which is a valuable prize, while the other two doors hide goats. After the contestant selects a door, the host, Monty Hall, who knows what is behind each door, reveals one of the remaining doors that hides a goat.
The contestant is then given a chance to switch their initial choice or stick with it. Surprisingly, statistical analysis demonstrates that switching doors increases the contestant’s chances of winning the prize. In this blog post, we will explore the Monty Hall Problem and provide a JavaScript solution to simulate the game and demonstrate the optimal strategy
Understanding the Problem
To comprehend the Monty Hall Problem, let’s break it down step by step
- The contestant selects one out of three doors (let’s call it the
initial choice
) - Monty, who knows the contents behind each door, intentionally reveals a door with a goat
- At this point, the contestant is given a chance to switch their initial choice or stick with it
- Finally, the contents behind the chosen door are revealed, and the contestant wins or loses
The key point to note is that Monty’s decision to reveal a door with a goat increases the contestant’s chances of winning if they switch doors after the revelation
JavaScript Implementation
Now, let’s dive into the JavaScript code that simulates the Monty Hall Problem and calculates the winning probabilities
// Function to simulate the Monty Hall Problem
function simulateMontyHall(numIterations) {
let switchWins = 0;
let stayWins = 0;
for (let i = 0; i < numIterations; i++) {
// Place the prize behind a random door
const prizeDoor = Math.floor(Math.random() * 3);
// Contestant's initial choice
const initialChoice = Math.floor(Math.random() * 3);
// Monty reveals a door with a goat
let revealedDoor;
do {
revealedDoor = Math.floor(Math.random() * 3);
} while (revealedDoor === initialChoice || revealedDoor === prizeDoor);
// Contestant switches the choice
const switchedChoice = [0, 1, 2].filter(
(door) => door !== initialChoice && door !== revealedDoor
)[0];
// Check if switching wins the prize
if (switchedChoice === prizeDoor) {
switchWins++;
} else {
stayWins++;
}
}
// Calculate winning probabilities
const switchWinPercentage = (switchWins / numIterations) * 100;
const stayWinPercentage = (stayWins / numIterations) * 100;
// Output the results
console.log(`Switching wins: ${switchWinPercentage.toFixed(2)}%`);
console.log(`Staying wins: ${stayWinPercentage.toFixed(2)}%`);
}
// Simulate the Monty Hall Problem with 10,000 iterations
simulateMontyHall(10000);
Explanation
The simulateMontyHall
function takes an argument numIterations
, which represents the number of times we want to simulate the game. Inside the function, we initialize variables to count the number of wins for switching (switchWins
) and staying (stayWins
)
The simulation is performed using a for
loop that iterates numIterations
times. In each iteration, we randomly assign the prize behind one of the doors, the contestant selects an initial choice, and Monty reveals a door with a goat. The contestant then switches their choice to the remaining unopened door. If the switched choice matches the door with the prize, we increment the switchWins
counter; otherwise, we increment the stayWins
counter
After the loop completes, we calculate the winning probabilities for switching and staying. These percentages are then displayed using console.log()
Conclusion
By running the JavaScript code provided above, you can simulate the Monty Hall Problem and observe the winning probabilities for both switching and staying. You will find that switching doors will yield a higher winning percentage in the long run, which defies the intuition of many people. The Monty Hall Problem serves as a captivating illustration of how probabilities can challenge our initial instincts and reveal the power of statistical analysis
Remember, the Monty Hall Problem is not merely a matter of chance but a mathematical phenomenon rooted in probability theory